class: center, middle, inverse, title-slide # Data Visualization and Design ## Using ggplot2
### Omni Analytics Group --- class: center, middle # POLISHING PLOTS --- class: inverse, center, middle # Visual Appearance --- ## Built-In Themes 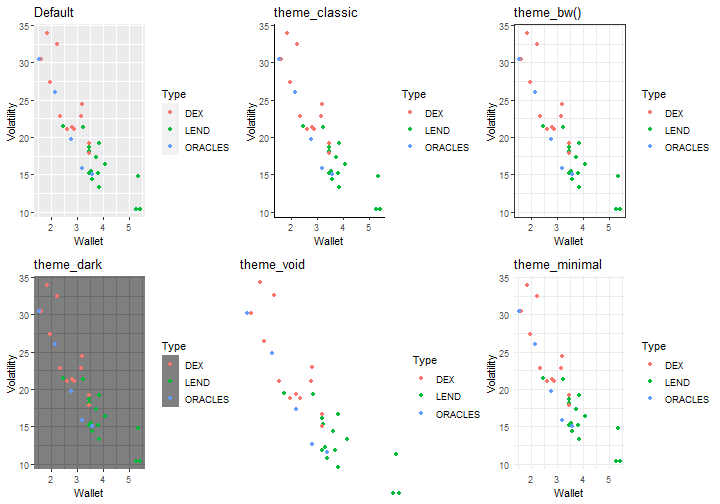<!-- --> --- ## Other Themes: ggthemes a ggplot2 extension 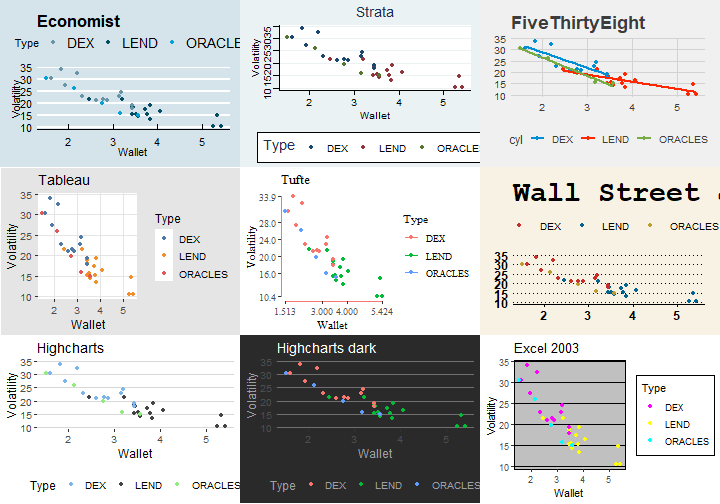<!-- --> --- ## Setting Themes You can globally set a theme with the `theme_set()` function: ```r theme_set(theme_bw()) ggplot(df, aes(x = Wallet, y = Volatility, colour = Type)) + geom_point() ``` 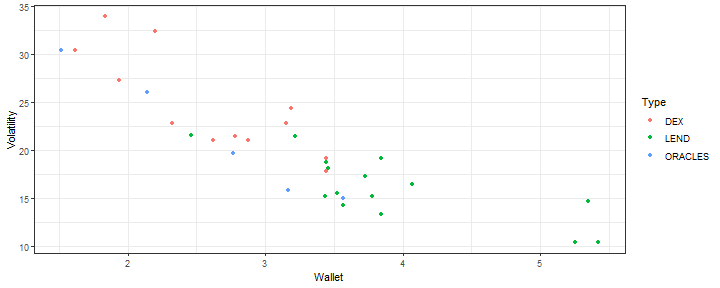<!-- --> --- ## Elements in a theme The function `theme()` is used to control non-data parts of the graph including: - **Line elements**: axis lines, minor and major grid lines, plot panel border, axis ticks background color, etc. - **Text elements**: plot title, axis titles, legend title and text, axis tick mark labels, etc. - **Rectangle elements**: plot background, panel background, legend background, etc. There is a specific function to modify each of these three elements : - `element_line()` to modify the line elements of the theme - `element_text()` to modify the text elements - `element_rect()` to change the appearance of the rectangle elements - `element_blank()` to draw nothing and assign no space **Note**: `rel()` is used to specify sizes relative to the parent, `margins()` is used to specify the margins of elements. --- ## Modifying a plot ```r p1 <- ggplot(punks) + geom_bar(aes(x = Type, colour = TypeSkin, fill = TypeSkin)) p2 <- p1 + theme_classic() + theme( ## modify plot background plot.background = element_rect(fill = "lightskyblue1",colour = "pink",size = 0.5, linetype = "longdash") ) ``` 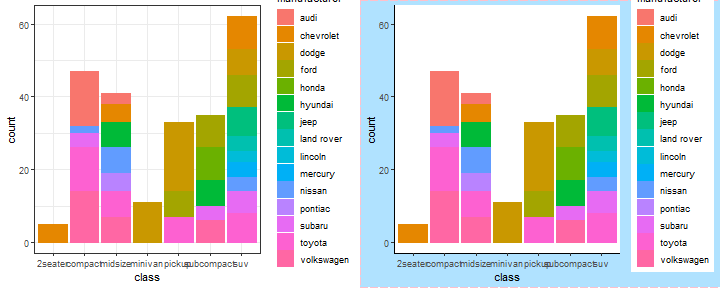<!-- --> --- ## Plot Legends ```r p3 <- p2 + theme( ### move and modify legend legend.title = element_blank(), legend.position = "top", legend.key = element_rect(fill = "lightskyblue1", color = "lightskyblue1"), legend.background = element_rect( fill = "lightskyblue1",color = "pink", size = 0.5,linetype = "longdash") ) ``` 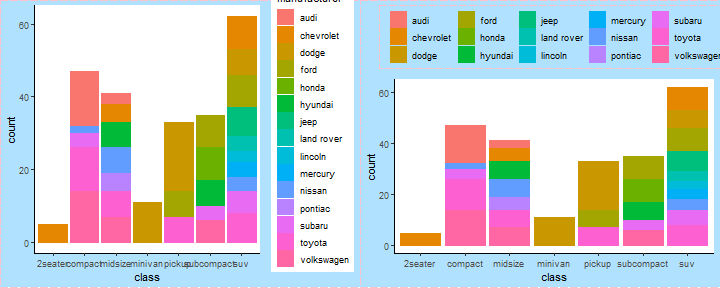<!-- --> --- ## Modifying Axes ```r p4 <- p3 + theme( ### remove axis ticks axis.ticks=element_blank(), ### modify axis lines axis.line.y = element_line(colour = "pink", size = 1, linetype = "dashed"), axis.line.x = element_line(colour = "pink", size = 1.2, linetype = "dashed")) ``` 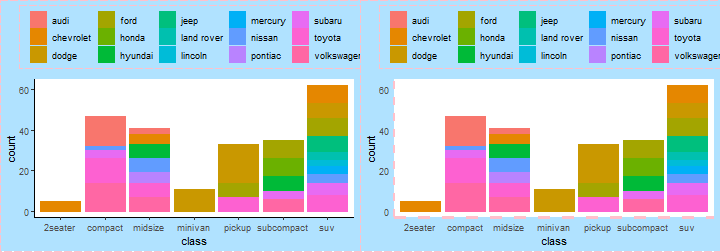<!-- --> --- ## Plot Labels Can be modified in several ways: - `labs()`, `xlab()`, `ylab()`, `ggtitle()` - You can also set axis and legend labels in the individual scales (using the first argument, the name) . ```r p5 <- p4 + labs(x = "Class of car", y = "", title = "Cars by class and manufacturer", subtitle = "With a custom theme!!") ``` 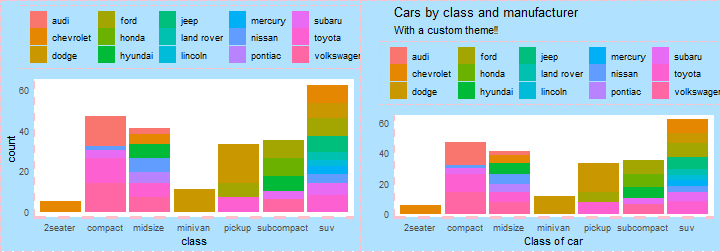<!-- --> --- ## Zooming ```r p <- ggplot(df, aes(x = Wallet, y = Volatility, colour = Type)) + geom_point() p_zoom_in <- p + xlim(2, 4) + ylim(10, 25) p_zoom_out <- p + xlim(0,7) + ylim(0, 45) ``` 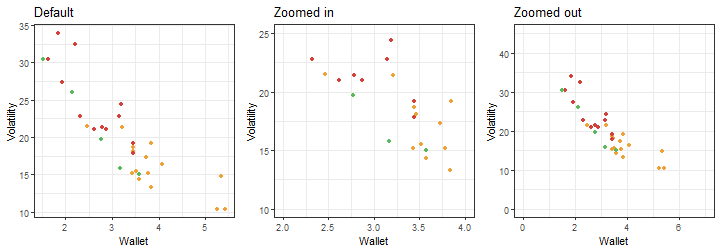<!-- --> --- class: inverse, center, middle # Interactive graphics --- ## Plotly ```r p <- ggplot(df, aes(x = Wallet, y = Volatility, colour = Type)) + geom_point() + scale_color_locuszoom() library(plotly) ggplotly(p) ```
--- class: inverse, center, middle # Saving graphics --- ## Saving your Work We can save the results of a plot to a file (as an image) using the `ggsave()` function: ```r p1 <- ggplot(df, aes(x = Wallet, y = Volatility, colour = Type)) + geom_point() ggsave("Volatilify_by_wallet.pdf", plot = p1) ``` <br><br> <p align="center"> <img src="images/Cut_outs/Cut_out_08.png" width="200px" height="160px"> </p> --- ## Your Turn 1. Create a scatterplot of End.Price versus Start.Price from the `cryptoart` data, colored by Platform 2. Use the black and white theme 3. Include an informative title 4. Move the legend to the bottom 5. Save your plot to a pdf file and open it in a pdf viewer. ```r ggplot(data = cryptoart, aes(x = Start.Price, y = End.Price)) ``` 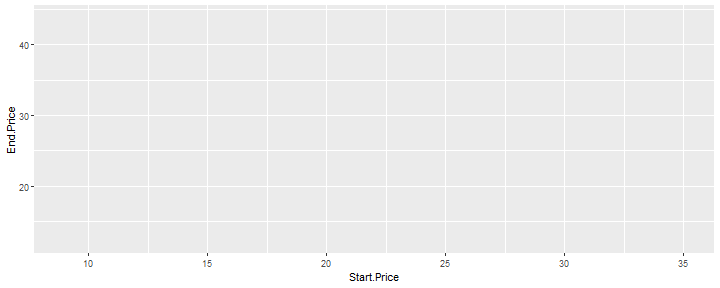<!-- --> --- ## Answers ```r p1 <- ggplot(data = cryptoart, aes(x = Start.Price, y = End.Price, colour = Platform)) + geom_point() + ggtitle("End Price vs Start Price by Platform") + theme( legend.position = "bottom" ) p1 ggsave("yourturn.pdf", plot = p1) ```